I’ve been working with the Pure1 REST for about a year now and have really enjoyed what it brings. I’ve integrated it into a few things: PowerShell. vRO. vSphere Plugin. One of the “tricky” things about it though is the authentication. Instead of a username and password it requires the use of a RSA256 public/private key pair. This is inherently more secure, but of course requires a bit more know-how when it comes to pair generation.
I simplified a fair amount of it in PowerShell, but didn’t quite get to the finish line. The generation of the key pair could be done but it came in the form of a PFX–which basically combines the public key and private key into one file. Unfortunately, Pure1 requires the them to be separated as all it needs is the public key, not your private key. While this is “better” it does leave Windows users at a bit of a disadvantage–there is no built in mechanism to generate this without installing OpenSSL directly. The process could not be done entirely in PowerShell. Or so I thought…
I blogged about creating the right type of certificate here. But you still need to extract the public key, which by default is not in the right format. This is where using OpenSSL came in, it had the ability to convert it into the PEM (good explanation of it here) which needs it to be encoded as INT64, not bytes, not hex, and certainly not decimal.
The requirement to install OpenSSL wasn’t a big deal I suppose as it was a one time kind of thing, but it was annoying. When we released support for Pure1 authentication in the vSphere Plugin recently it came up again (in my mind at least). How can we simplify this? First off, lets review the steps to authenticate for Pure1:
- Generate a RSA256 public/private key.
- Add the public key to Pure1.
- Pure1 gives you an application ID.
- With that application ID, generate a JSON web token that is encrypted with your private key. You can specify in that token how long it is valid for, in other words for how long it can be used to create a session with Pure1.
- Send the JWT to Pure1.
- Pure1 sends you back a session token. That token is valid for 10 hours (or until you remove the public key from Pure1–whichever comes first.
Our vSphere Plugin asks you for a JWT. So you generate a JWT configure it in the vSphere Plugin:
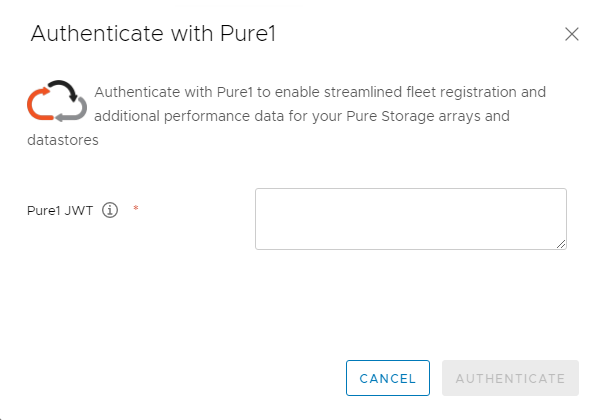
So how do I get to that?! Well I just made it A LOT easier. I have updated my Pure1 PowerShell module with a few new cmdlets.
- New-PureOneCertificate
- Get-PureOnePublicKey
- New-PureOneJwt
Authenticating Pure1 with the vSphere Plugin
Let us start with the vSphere Plugin. How do I get that infernal JWT? Good question Cody.
Step one is to install the module. So either run:
install-module PureStorage.Pure1
Or if it is already installed:
update-module PureStorage.Pure1
NOTE: If you already have the Cody.PureStorage.Pure1 module installed uninstall it first and then install this one. While the Cody.PureStorage.Pure1 version has this feature, I am deprecating it. Also if you are using the PureStorage.FlashArray.VMware module, make sure you update it first too, as older versions have a dependency on the Cody.PureStorage.Pure1 module.
Now let’s create a new certificate.
$cert = New-PureOneCertificate
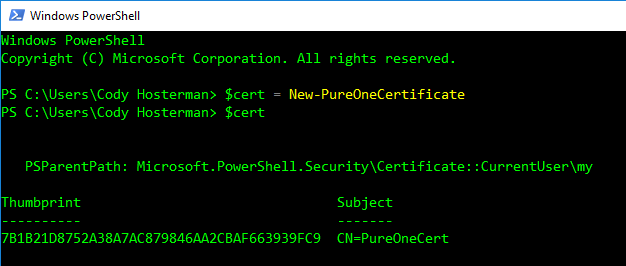
Btw, if you are using Windows 2012 R2, make sure you are at least on the 1.0.0.1 version of the module.
By default I create it in the current user store (which incidentally makes it not run into this problem anymore). Though there is a parameter to override that with a certificate store of your choosing.
Great. Now I need to add the public key from this certificate to Pure1. So run:
Get-PureOnePublicKey -certificate $cert

Now copy the result of that (including the dashes and public key stuff). Highlight it and press Enter.

I will write a blog post on how I did this without installing OpenSSL soon.
Now got to Pure1.purestorage.com and click on API Registration:
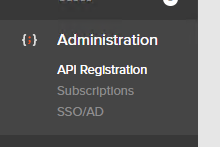
If you do not see this, that means you are not a Pure1 admin. Find your admin. Or become one. Click on Register Application.
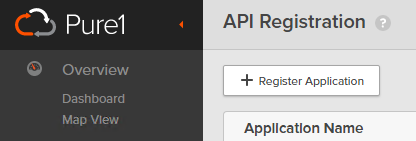
Now paste that public key into that.
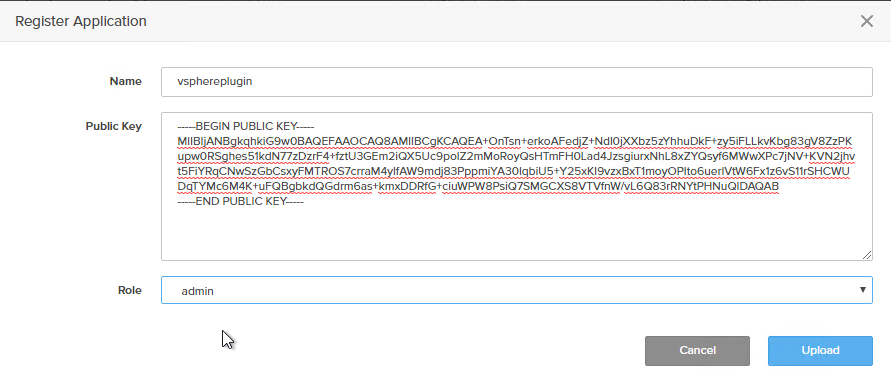
Give it a name and choose a role. Currently this is admin or read only.
The only significant difference that I am aware of is that a read only user cannot create tags. Beyond that everything else is currently read only. But this is 12/03/2019. This may and WILL change in the future, admin will likely be able to do more things in the future.
Click Upload.
You will no longer need to do this again, unless you want to create a separate key for something else. Or you lose/delete the private key/certificate.
You will see it listed now. Copy the application ID.

It will be in the form of pure1:apikey:………….
Back to PowerShell. Run:
New-PureOneJwt -certificate $cert -pureAppID pure1:apikey:rUMmI6I7LEneeAfR

Don’t worry, this public key has been deleted by the time of publishing. You can’t use it :). Nice try though.
So how long does this JWT authorize the plugin to talk to Pure1 for? Not forever. One of the internal fields of the JWT is an expiration date. This date says that this JWT can be used to create new Pure1 REST sessions until this date in time OR you can remove the public key from Pure1–this will kill the use of any JWTs for that key entirely.
The default behavior of the new-pureonejwt cmdlet is to create a JWT that is valid for 30 days. Though you likely want longer than that. The cmdlet has a parameter called expiration that allows you to specify a custom expiration date too.It takes in the dateTime data type.
So if you want it to last a year, specify a date one year in the future. Which would be like (Get-Date).addYears(1). If you want 60 days: ( (Get-Date).addDay(60) )
New-PureOneJwt -certificate $cert -pureAppID pure1:apikey:rUMmI6I7LEneeAfR -expiration((Get-Date).AddDays(60))

Copy that full response and go to the Pure Storage vSphere Plugin home screen and click on Authenticate with Pure1.
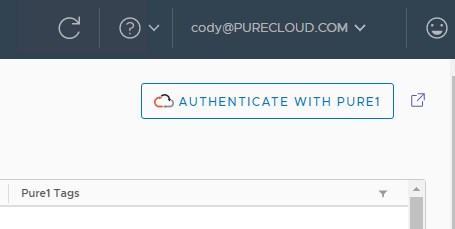
Paste the JWT into there
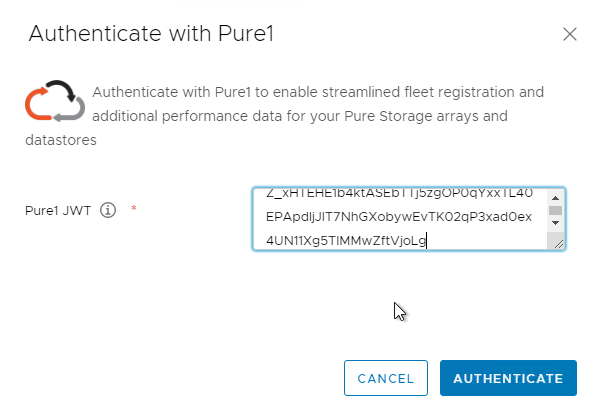
Click Authenticate.
You’re done!
If this fails, check out this post:
Authenticating PowerShell with Pure1
What if I want to authenticate the Pure1 PowerShell module with Pure1? Well this is even easier then the above.
Now let’s create a new certificate.
$cert = New-PureOneCertificate
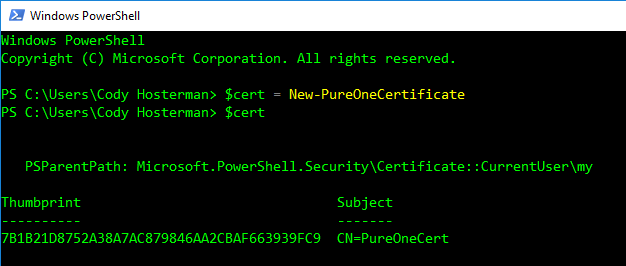
By default I create it in the current user store (which incidentally makes it not run into this problem anymore). Though there is a parameter to override that with a certificate store of your choosing.
Great. Now I need to add the public key from this certificate to Pure1. So run:
Get-PureOnePublicKey -certificate $cert

Now copy the result of that (including the dashes and public key stuff). Highlight it and press Enter.

Now got to Pure1.purestorage.com and click on API Registration:
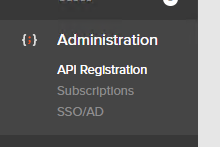
If you do not see this, that means you are not a Pure1 admin. Find your admin. Or become one. Click on Register Application.
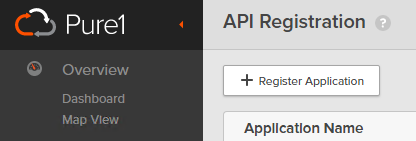
Now paste that public key into that.
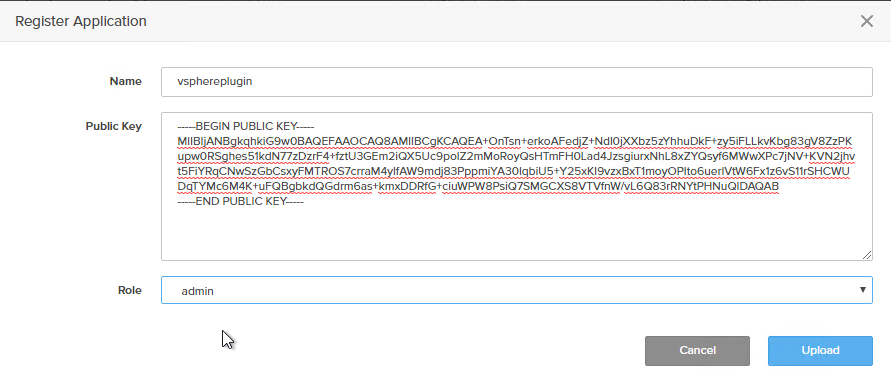
Give it a name and choose a role. Currently this is admin or read only.
The only significant difference that I am aware of is that a read only user cannot create tags. Beyond that everything else is currently read only. But this is 12/03/2019. This may and WILL change in the future, admin will likely be able to do more things in the future.
Click Upload.
You will no longer need to do this again, unless you want to create a separate key for something else. Or you lose/delete the private key/certificate.
You will see it listed now. Copy the application ID.

It will be in the form of pure1:apikey:………….
Back to PowerShell. Run:
New-PureOneRestConnection -certificate $cert -pureAppID pure1:apikey:rUMmI6I7LEneeAfR
The session is authenticated and you can now run commands against Pure1.

Like Get-PureOneArray
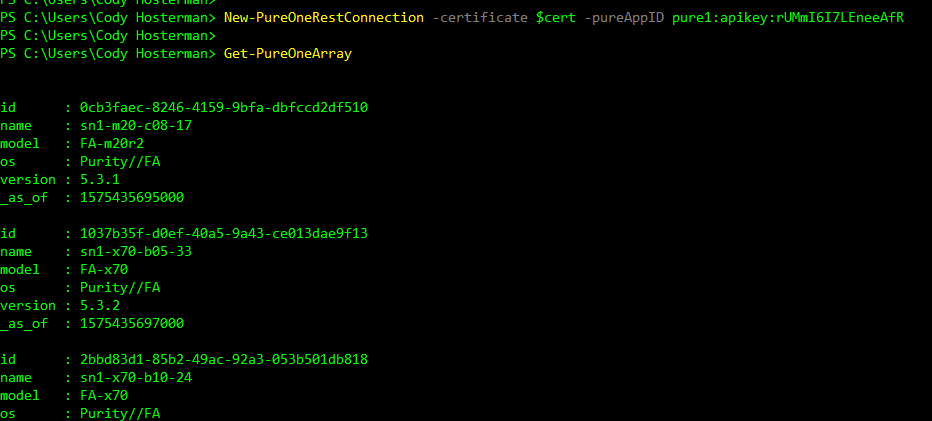
Thank you for making this much easier after spending 3-4 hours going through step by step in your previous blogs. Learned lots but thinking to myself how many people would really do this if it wasnt made easier. Scott
Ugh sorry about that… Wished I was a bit quicker on the draw. Walk through this and let me know if you have feedback. Should be MUCH easier.
Ok – I normally do not use windows for anything and just found out about powershell this year. Which means I am way behind the curve. Is there anything like “get to know terms” – “Cheat sheet on what things are” — “helpful dictionary terms”.
What is REST API – what do I want to do with it? Why do I need to have it?
What is RSA256 public/private authentication, I know what and how to set that up in *NIX land, but, not windows.
Can any of this be done in *nix land? I have lots of *nix servers that I can log into and point at the pure storage arrays, I only have my work laptop with windows to play with powershell.
Please, before I get the normal “Google is your friend flaming posts” Who has time to go through thousands of hits from google and try and do the right keywords thus getting close to what you want. I am extremely busy with hundreds of other things that need to be installed and fixed or changed for our environments. Just do not have time to fiddle around with powershell.
Bill,
If you are a mostly Linux shop PowerShell might not be the best route for you. PowerShell does run in Linux now, but if you don’t have experience in it, I would probably suggest Python as an orchestration route. This is far more popular in non-Windows environments. Plus the openssl options built into Linux is the best way to do the whole public/private key route. While you can script against a FlashArray (or FlashBlade) with SSH, it requires a fair amount of test parsing–REST based responses are returned in JSON, so it is much easier to handle then text blobs. Pure1 doesn’t have a CLI so to get information out of it you would need to use REST for sure. You dont have to use PowerShell though, you can use Python or many other tools (or even curl if you so choose). The short answer though is if you just want to run a few command against an array and just see the response, there isnt a significant advantage to REST over SSH (CLI) but if you want to orchestrate workflows, provisioning or deliver metrics to some monitoring application (etc) REST is a much better option–especially since APIs are guaranteed to be backwards compatible and not introduce breaking changes. There are no such guarantees with SSH/CLI. I blog a lot about PowerShell because it is very popular in VMware environments, but going that route is by no means the only correct option. I’d start with figuring out what you want to do, then choose the right tool.
This was very timely, thank you, but I am running into an issue. I’m new to this, so if this is a rookie mistake, please be kind. ๐ I made sure I had the latest PureStoragePowerShellSDK and PureStorage.Pure1 modules installed. But when I run the cert command I get this error:
PS C:\Users\!tborcher> $cert = New-PureOneCertificate
New-SelfSignedCertificate : A parameter cannot be found that matches parameter name ‘HashAlgorithm’.
At C:\Program Files\WindowsPowerShell\Modules\PureStorage.Pure1\1.0.0.0\PureStorage.Pure1.psm1:40 char:79
+ … rtificate -certstorelocation $certificateStore -HashAlgorithm “SHA256 …
+ ~~~~~~~~~~~~~~
+ CategoryInfo : InvalidArgument: (:) [New-SelfSignedCertificate], ParameterBindingException
+ FullyQualifiedErrorId : NamedParameterNotFound,Microsoft.CertificateServices.Commands.NewSelfSignedCertificateCo
mmand
I searched for other Pure Storage modules I might need and installed PureStorage.FlashArray.VMware* as well. Same error. What am I missing? I know it’s got to be something basic. Thanks.
What version of PowerShell are you on? I believe this parameter was introduced in PowerShell 5.
PS C:\Users\!tborcher> get-host|select-object version
Version
——-
5.1.14409.1018
This is on a Windows 2012 R2 server. Looking at Microsoft Windows 10 / 2016 docs (https://docs.microsoft.com/en-us/powershell/module/pkiclient/new-selfsignedcertificate?view=win10-ps) it shows this HashAlgorithm option in Windows 10 / 2016, but not in Windows 2012 R2 (https://docs.microsoft.com/en-us/powershell/module/pkiclient/new-selfsignedcertificate?view=winserver2012r2-ps).
So I found a 2016 Server and all the commands worked fine there. However, the final step of authenticating from within vCenter is not working. Both I and my colleague, using different servers to build the key and registering different “Applications” in Pure1 and authenticating from different vCenters got the same error:
Error validating token. An unknown error occurred trying to reach Pure1.
I am about to hop on a flight back to SF, I should be in the office around 2:30 or so. Want to have get on a zoom and I can look at it with you?
BTW I think I figured out a workaround for 2012 that will allow me to support that release too, but i need to test it. Just requires some .NET work if I detect that OS version
Unfortunately I cannot do a Zoom tonight (5:30 ET). If you are available at 11AM ET (8AM PT) on Friday, I could meet then. Or we can continue the conversation here or via email. Thanks!
Hi Cody
I have no such thing as a menu called “administration” in pure1 – do i need some kind of special access? (we are a ASP)
You need to be an administrator in your org. Sounds like your login is not an admin.
Any support for PS on MAC?
Iโve never tested this on Macs but I donโt think it will work (at least not the authentication portion.) Id recommend using the Python toolkit for Macs that should work fine
…or when cert expires in 1 year?
> You will no longer need to do this again, unless you want to create a separate key for something else. Or you lose/delete the private key/certificate.
If it is a Windows-based cert, maybe though I am not sure if the certificate expiring precludes it from being used to create a signed JWT. Yes certainly if the JWT expires the JWT will need to be regenerated.
It works so good, also for “not powershell guru” ๐
Thanks a lot