I just posted about some new cmdlets here:
Also in that release are a few more cmdlets concerning storage policy creation, editing, and assignment. They were built to make the process easier–the original cmdlets and their use is certainly an option–and for very specific things you might want to do they might be necessary, but the vast majority of common operations can be more easily achieved with these.
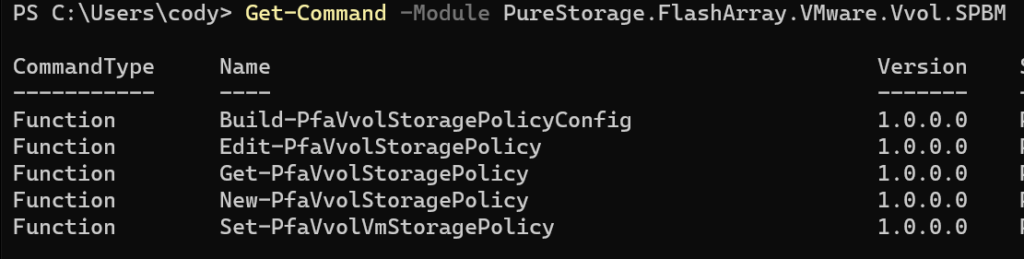
As always, to install run:
Install-Module PureStorage.FlashArray.VMware
Or to upgrade:
Update-Module PureStorage.FlashArray.VMware
These modules are open source, so if you just want to use my code or open an RFE or issue go here:
https://github.com/PureStorage-OpenConnect/PureStorage.FlashArray.VMware/
For detailed help on a cmdlet, run Get-Help
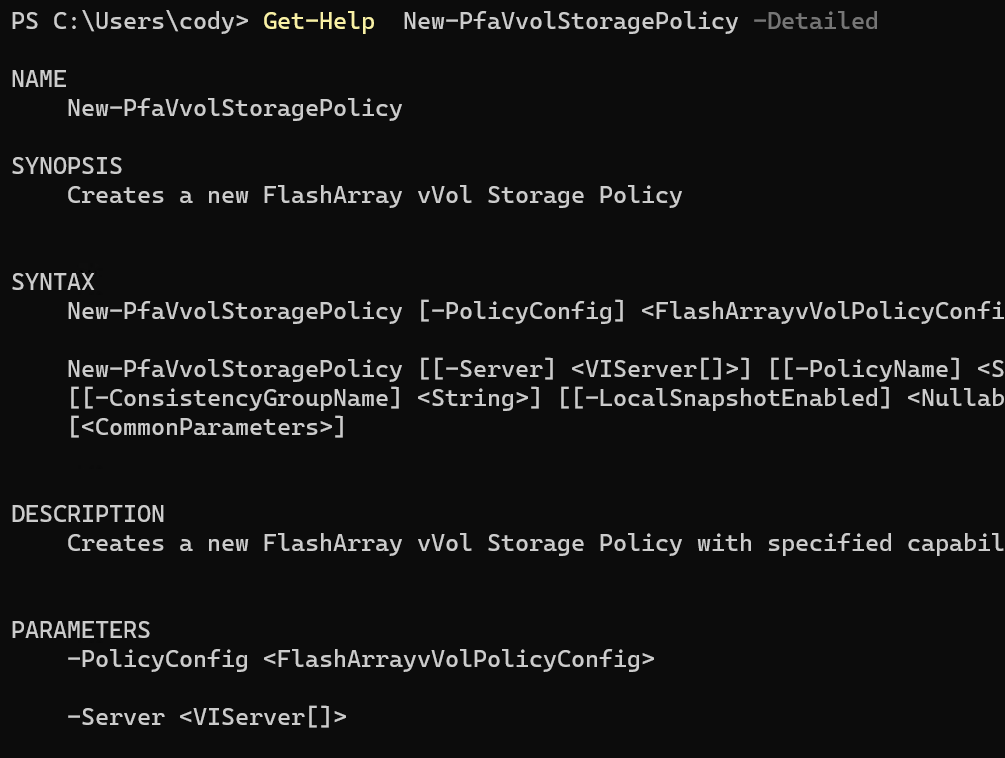
I have written about assigning storage policies in the past in this series:
But the process may not be super intuitive or at the last is kind of TOO flexible. Too many options and steps that aren’t needed for most people. So these cmdlets are gauged at the more common operations.
Get-PfaVvolStoragePolicy
This returns any storage policy that includes one or more Pure Storage capabilities:
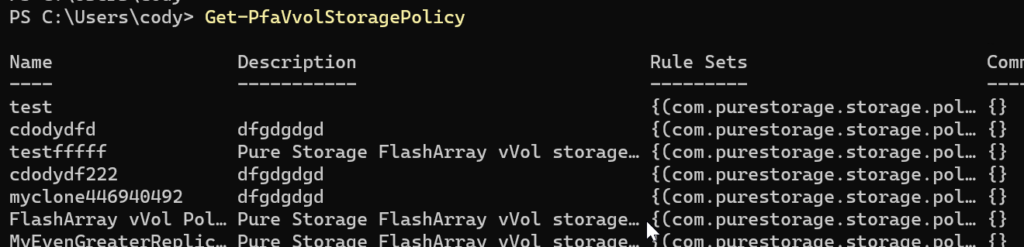
It is like Get-SpbmStoragePolicy with a specific filter.
You can also include the -Replication parameter and it will only return policies that have replication vVol features in them.

Lastly you can narrow down by policy name and/or by vCenter server if you are connected to more than one.
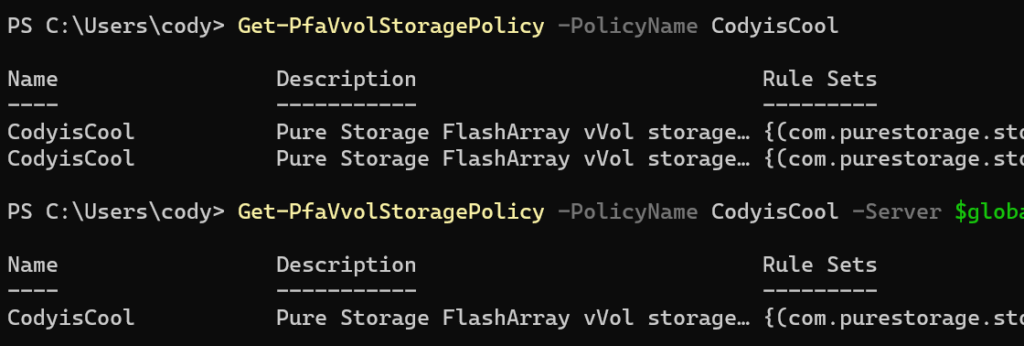
Storage policy names are unique to a vCenter, not globally.
Build-PfaVvolStoragePolicyConfig
This cmdlet does a simple thing–it creates a PowerShell object that can be used to create a Pure Storage vVol SPBM policy. You run it and it will return an object with all of our vVol capabilities you can then populate with your desired configuration.
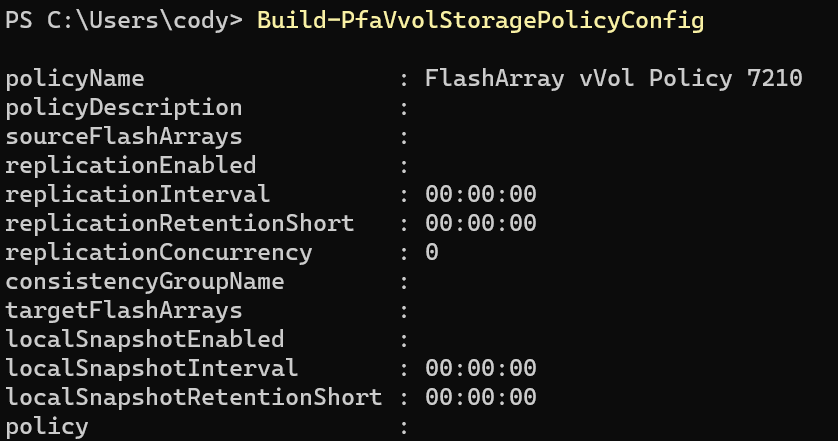
It will create a default name but you can change any of these properties. So if I want to create a default policy with the name CodyisCool:
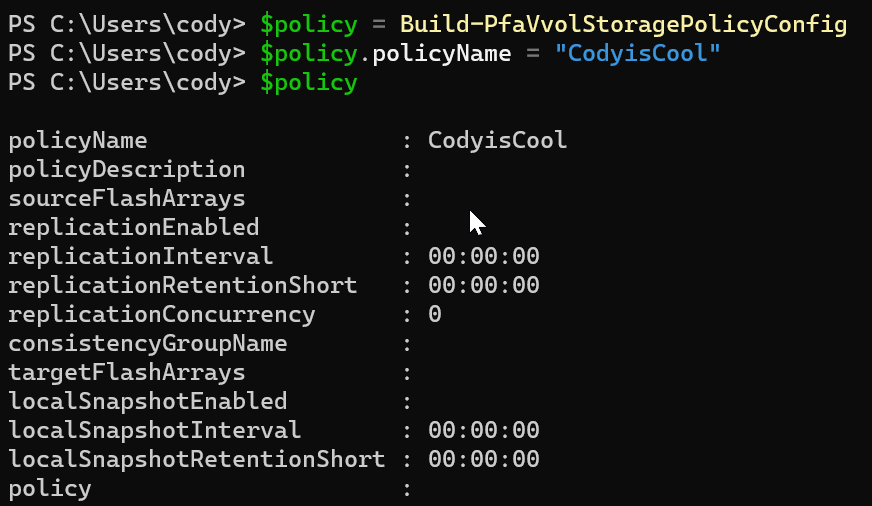
If I were to create this policy it would only have one capability enabled which is says anything under this policy should be on Pure vVols:
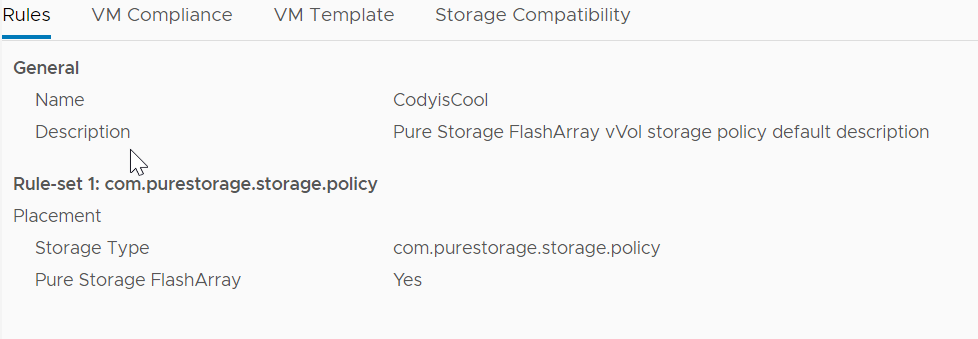
Additionally, you can use this cmdlet to pull a config from an existing policy. So if I have the following policy:
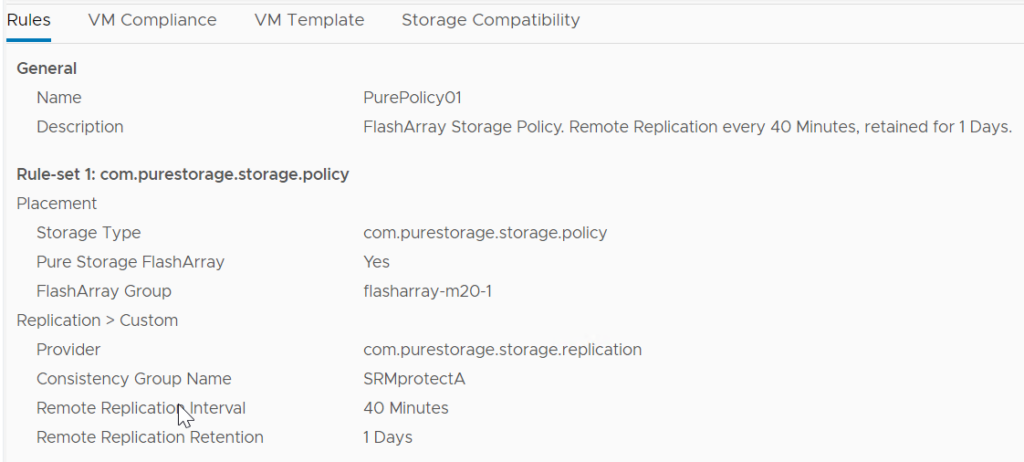
And I want to edit it, or clone it, I can pass it into this cmdlet and it will create the corresponding object:
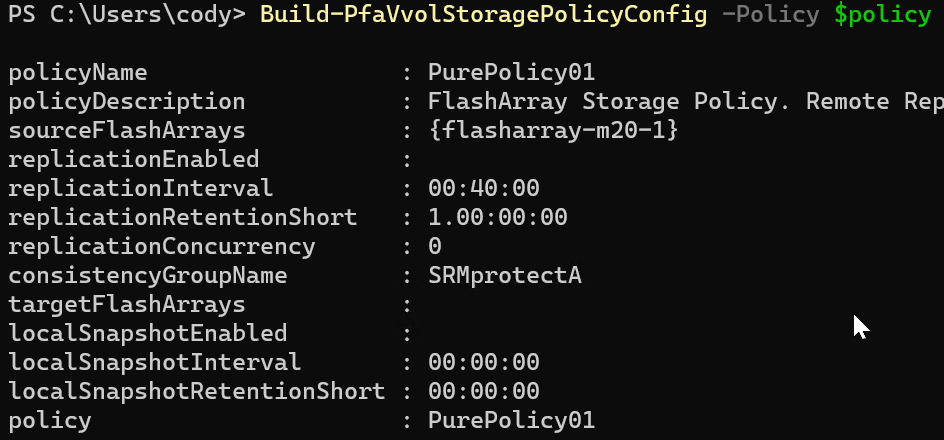
You might notice that the new object also stores a reference the policy in the policy property:

I can then take that and edit the object and create a new policy or edit the original one by applying my changes. Let’s edit the existing one first.
Edit-PfaVvolStoragePolicy
In the previous step, we took an existing policy, called PurePolicy01 and created an object config from it.
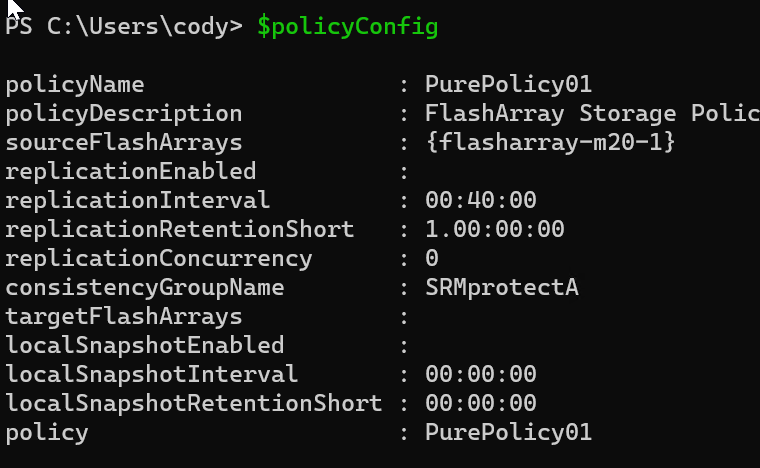
Let’s review what this policy dictates right now, it says any vVol provisioned under it must:
- Be on a FlashArray
- Be on a specific FlashArray called flasharray-m20-1
- Be replicated every 40 minutes
- Each replication point-in-time must be kept on the target for 1 day then deleted
- Be in a replication consistency group called SRMprotectA
All of the other capabilities are not specified and can either be not configured or configured–the policy is not configured to require them to be on/off or set in a certain way. Now I want to make some changes to the policy. Currently, it says to replicate every 40 minutes and to keep each replication point-in-time (retention) for 1 day. Let’s make it replicate every 5 minutes and keep everything for 12 hours instead. Let’s also update the description to reflect the change.
Intervals are specified as a specific data type called a TimeSpan. This can be easily created with the New-TimeSpan cmdlet. So if I want to make the replication interval 5 minutes it would be this command:
$policyConfig.replicationInterval = New-TimeSpan -Minutes 5
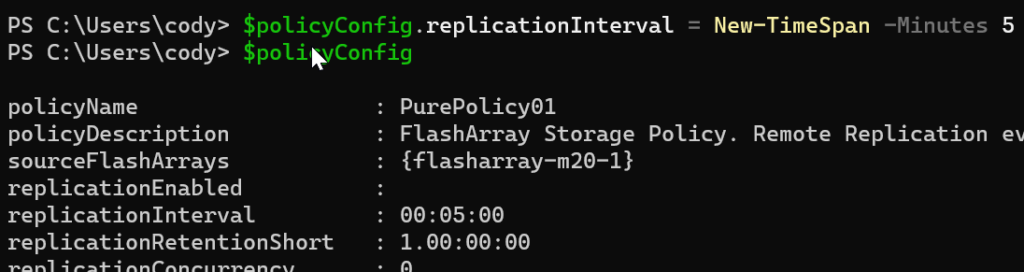
Same for the retention:
$policyConfig.replicationRetentionShort = New-TimeSpan -hours 12
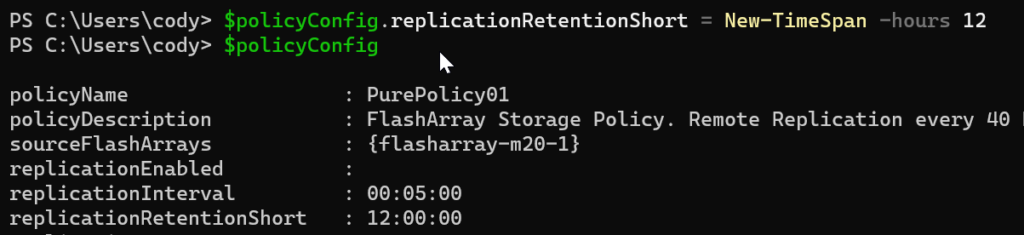
Now to fix the description which is simple text:

Now how to apply this? Run the Edit-PfaVvolStoragePolicy and pass in that object.

An voila!
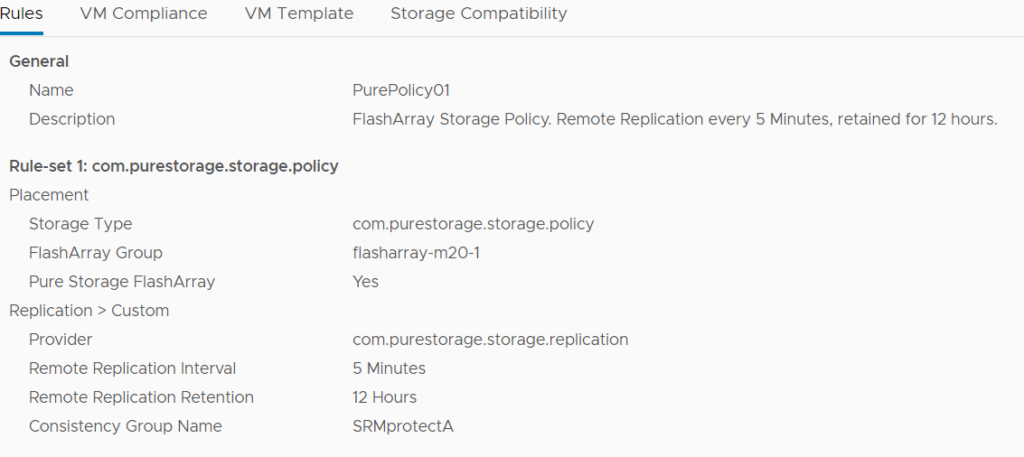
New-PfaVvolStoragePolicy
This is a very similar process as edit, except you do not need to pull in an existing policy. But you can if you want to–to use as a template.
You have two options here. Use Build-PfaVvolStoragePolicyConfig to create a template either one from scratch or from an existing policy following a similar process as described above but instead of passing the $policyConfig to Edit-PfavVolStoragePolicy you use New-PfaVvolStoragePolicy.

Alternatively, you can skip the config step and just create a new policy in a one-liner.

The default behavior is that it will create the policy on every vCenter currently authenticated in your PowerCLI session. You can narrow it down with the -Server parameter though.
The default policy will just say: “anything in this policy must be on Pure vVols”:
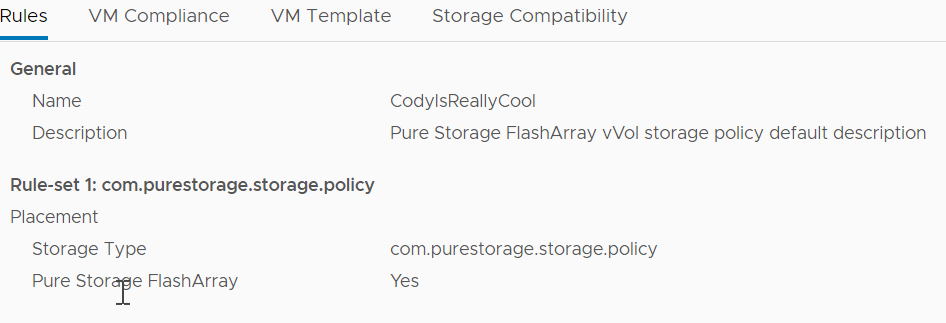
It will also assign the default description. But you can specify all of the values as shown above. Let’s set the FlashArray group value. This says: any vVol under this policy MUST be on one of the following arrays.
I want it to be on my m50-1 or my x50-1. The datatype it takes in is VASA storage arrays, which can be retrieved with Get-PfaVvolStorageArray:
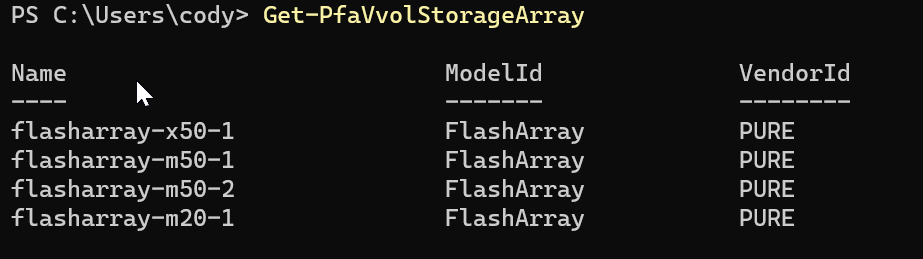
So the creation would look like:
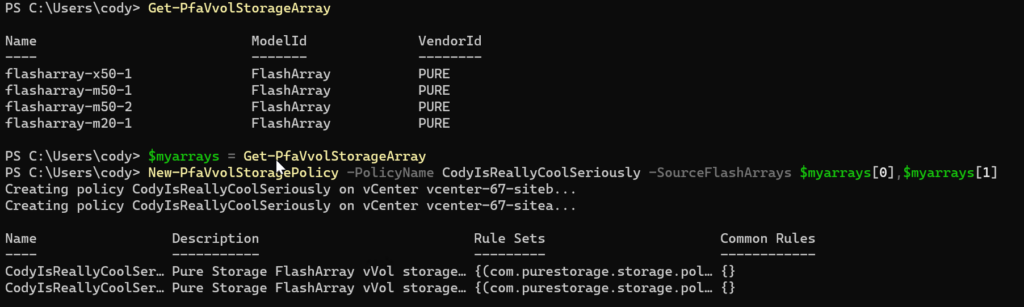
Done:
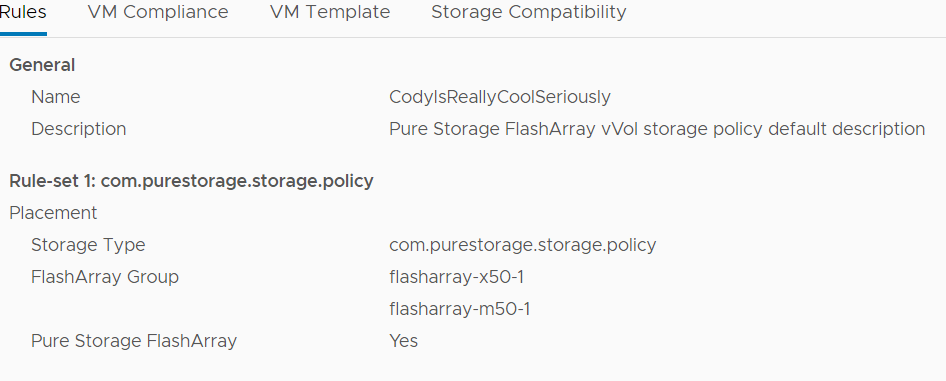
Set-PfaVvolVMStoragePolicy
Now that I have created a policy, I want to assign it. This can be done through a few cmdlets like Get-SpbmEntityConfiguration and Set-SpbmEntityConfiguration as well as a few other operations demonstrated in the blog post linked earlier. This cmdlet streamlines this in favor of less options.
At a high level it:
- Takes in one or more VMs. These VMs must already be on a Pure vVol datastore–the cmdlet does not initiate a Storage vMotion to correct this for you.
- Takes in a policy
- If that policy is a replication type policy, the cmdlet requires the entry of a replication group. This can either be a specific one or an automatically created one. For replication policies it requires that all VMs be on the same vVol datastore that were passed into the cmdlet at once.
- It will then assign the policy and optionally the replication group. If automatic was chosen it will create a new replication group and then assign the new one to all VMs (their home vVol and all of their disks).
To assign a vanilla Pure Policy:

As you can see it just says “put this on Pure”
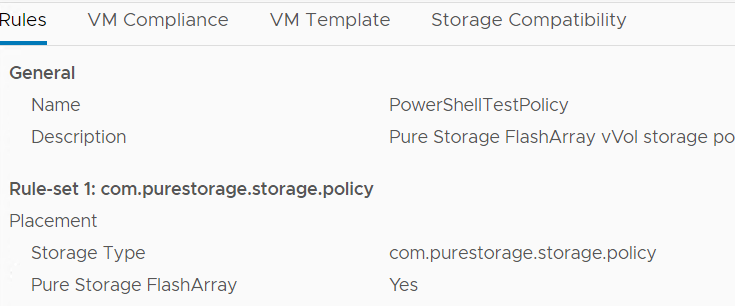
I want to assign it to a set of my VMs:
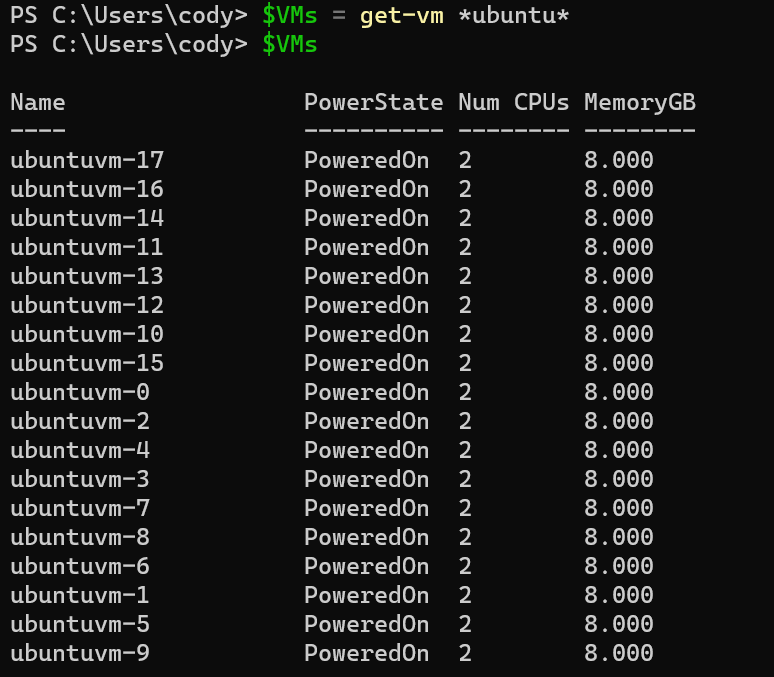
They currently have a policy assigned I don’t want.
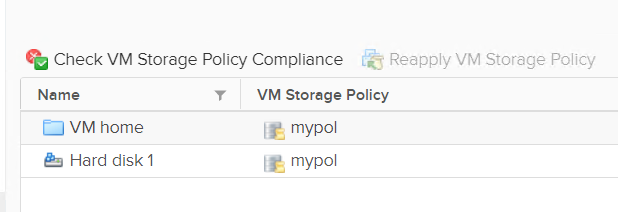
So let’s assign this policy:
Set-PfaVvolVmStoragePolicy -VM $VMs -Policy $newPolicy

You will see the VMs assigned the policy:
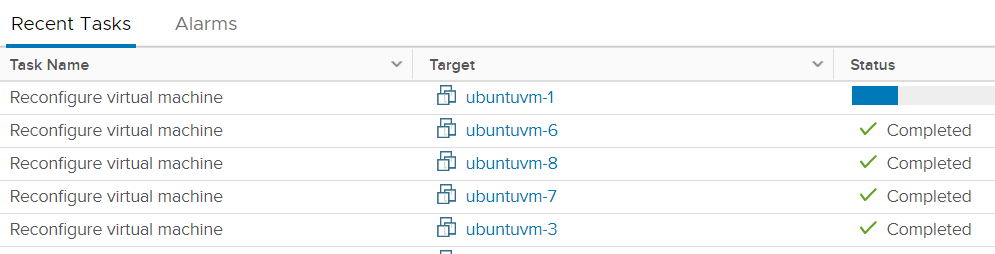
And when complete, it will return every object and its compliance status:
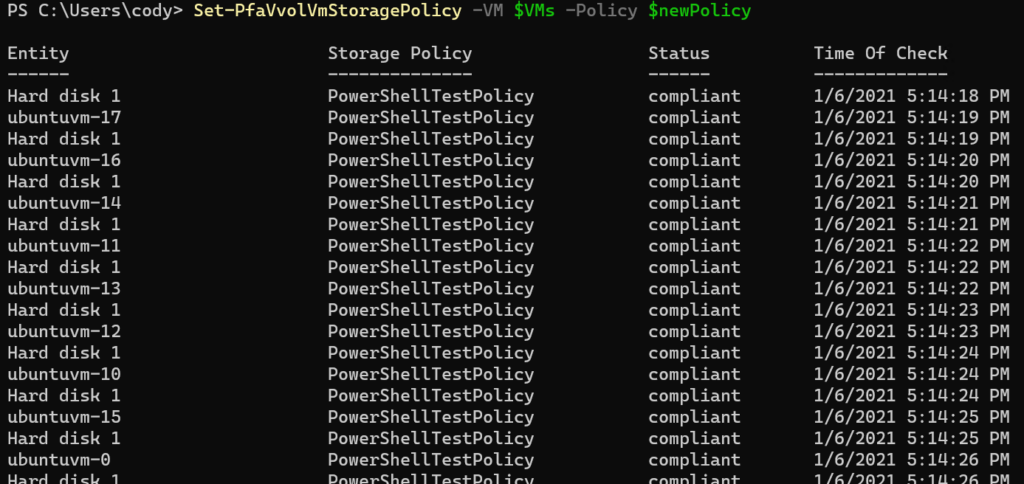
Assign with an automatic replication group
So let’s say I want to assign the following policy to my VMs:
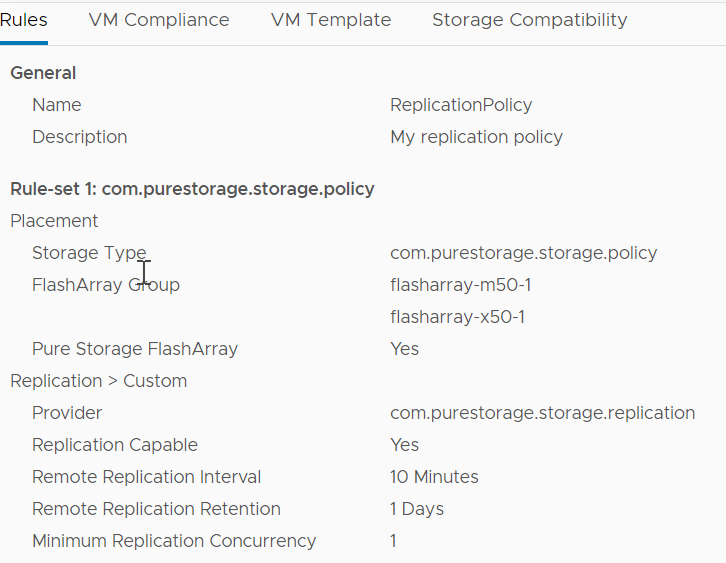
Store that in $Policy:

This is a replication policy so it requires a replication group. I don’t care what group–I just want them to be in the same one. So I can use -AutoReplicationGroup:
Set-PfaVvolVmStoragePolicy -VM $VMs -Policy $Policy -AutoReplicationGroup

This will cause a new protection group to be created on the FlashArray:

And those VMs to be assigned to that corresponding replication group:
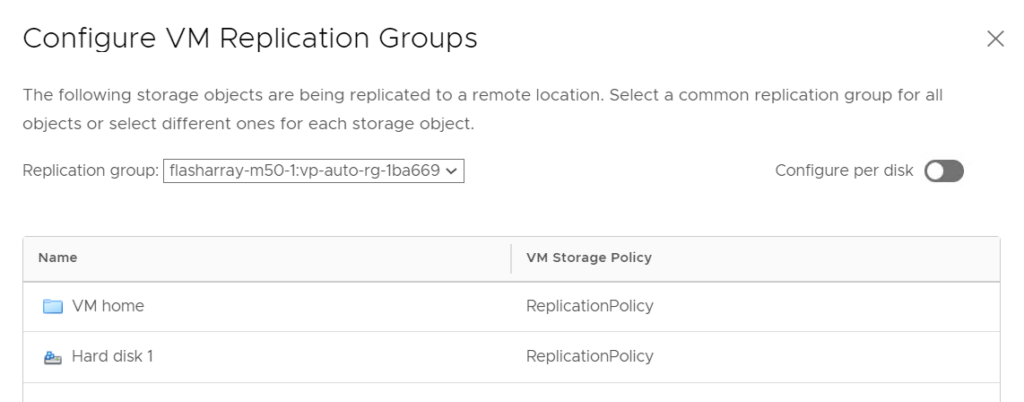
Assign with a specific replication group
Alternatively, you can pull the available compatible replication groups from the policy above:
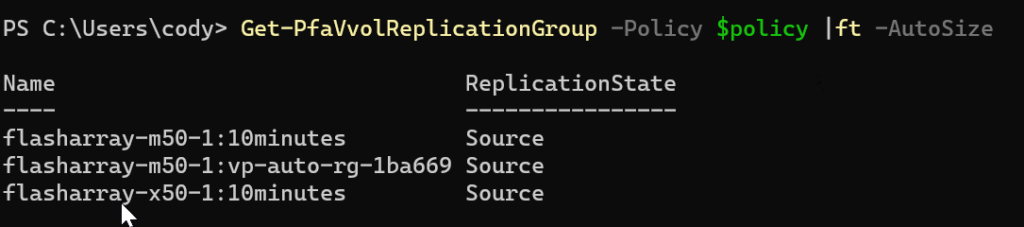
And then assign the policy with the desired group (I will put it in the first one in the list):

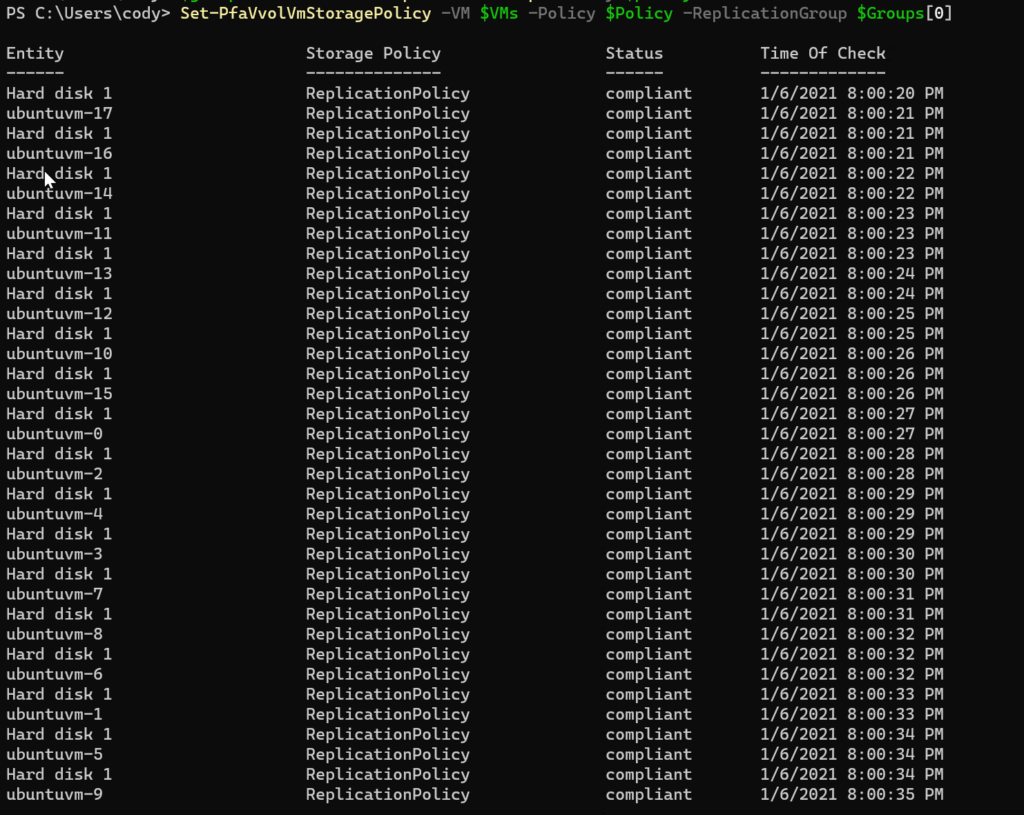
Final note–there were some fixes in PowerCLI so do make sure you are on 12.1 or later of PowerCLI.
How can a VM storage policy set a VM to be a part of multiple protection groups? Use case is a single array replicating to two arrays and wanting different retention policies per target.
Hi Matt,
Short answer is that with storage policies you can’t. As storage policies will only allow a single replication group to be associated with a VM (like even the same vm will get a pbm fault if you try to use different rg’s for each object).
If wanting to replicate to multiple targets with different sla’s, then this has to be manually done on the array. For example, you would need to create an additional protection group on the array with the different replication interval and retention, then manually add each config, data, etc to that protection group. Keep in mind that VASA will not add volumes to that pgroup automatically, so if VMs have managed snapshots or new virtual disks, those volumes won’t be added to that pgroup.
Sadly however, doing this and manually adding volumes to pgroups on the array will not allow you to leverage failover api’s to replication groups that are not associated with the storage profile and metadata on that VM. One other thing, I’ve found that VMware and SRM in particular do not like replication groups with multiple targets, so that’s something to avoid as well.
Not much of a short answer, but this is something Cody and I have discussed and have had ongoing conversations internally about what we can do to make situations such as your possible with vVols and SPBM. I don’t have a timeframe on when we’ll see that, but it is something we want to improve.